Collision Trace System
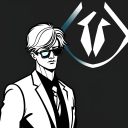
Overview
In simple terms, the GCS_CollisionSystemComponent
is a component used to manage multiple collision trace instances.
Each collision trace instance does one thing: it binds to a PrimitiveComponent
(collision shape, Mesh, etc.) and uses the GameplayTargetingSystem
to acquire, filter, and sort target Actors. Users can bind events on the collision trace instance to retrieve and process target results.
What Problems Does It Solve?
- Characters can bind two collision trace instances to their hands, enabling hand-based target detection. These instances can be activated and used to acquire targets when using punching/grabbing abilities.
- Projectiles can create collision trace instances based on their shape and use them to hit targets during flight.
- abilities can use these instances to acquire ability targets.
- The target detection logic can be dynamically adjusted based on activation time or ability level, such as varying the detection radius as activation time increases or decreases.
Gameplay Integration
Creating/Removing collision trace Instances

If your game features an elite Boss that uses both hands to grab player abilities, you should create two collision trace instances (using a spherical detection TargetingPreset
), named LeftHand
and RightHand
, during the character's BeginPlay
, and bind the Mesh to these instances. When activated, these instances will perform target detection centered around the left and right hand sockets.
Activating/Deactivating collision trace Instances

In GCS, you can use ANS_GCS_AttackTrace
to automatically activate/deactivate detection instances. The BP_GCS_Projectile
will also automatically activate/deactivate instances based on conditions, so you will rarely need to call these APIs directly.
Listening for Hit Targets

Collision trace Instance
It is a UObject
with its own lifecycle. It is managed by an object pool, ensuring high performance, and other systems in the game can call to activate or deactivate a detection instance at any time.
Detection Information (TraceInformation)
Each detection instance carries valid TraceInformation
when activated, which is a GameplayEventData
structure containing:
- Instigator: The source Actor that activated this instance (either the
AvatarActor
or a weapon/projectile). - OptionalObject: The attacking request object.
Other fields not listed are not currently used but may be utilized in the future.
Built-in collision trace instance Types

GCS provides three types of collision trace blueprints by default, which can meet most common needs:
ShapedBased requires that the incoming PrimitiveComponent be a ShapeComponent or one of its subclasses (Box, Capsule, and Sphere) and performs target detection based on this shape.
SocketBased requires the incoming PrimitiveComponent to be a Mesh with socket names specified by PrimitiveComponentSocketNames
and performs target detection based on the number and location of the sockets.
Adding new collision trace instance Types
You can create a subclass from GCS_CollisionTraceInstance
using Blueprints or C++.