Ability System Component
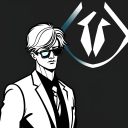
Ability System Component
The GGA_AbilitySystemComponent
is a built-in AbilitySystemComponent extension, and it is very lightweight.
Any actor only needs to add GGA_ AbilitySystemComponent to use GameplayAbility. You don't need to define the component for the character in C++.

Default settings
This component can configure multiple AbilitySets (see below) and also specify the Replication Mode for GameplayEffects.

GameplayTagRelationshipMapping
The component can also specify a data asset of type GGA_AbilityTagRelationshipMapping to define blocking, interrupting, and activation requirement relationships between different abilities. This mapping is referred to as TRM
This allows you to manage relationships between multiple abilities in one place, rather than defining relationships with other abilities in multiple distributed abilities. This can greatly simplify your management, especially if you have hundreds of Gameplay Abilities.

This picture is an example of AbilityTagRelationshipMapping (taken from my combat system)
At the same time, you can also switch to different mappings at runtime, making it easy to dynamically modify game mechanics.

For example, in the above TRM, backstep is blocked when character roll. What if your designer asks you to follow Roll after Step? At this time, dynamically switching TRM comes in handy.
Setup and Initialization
Check it for the original setup. https://github.com/tranek/GASDocumentation?tab=readme-ov-file#412-setup-and-initialization
You can call this method to initialize the ability system with lives on pawn. Internally, it will call InitAbilityActorInfo and grant default abilities (only execute on server side.)

And it's identical to this:

For client side, you can use a rpc to tell client execute the same code.

Understanding AvatarActor and OwnerActor
Typically, the Actor where an AbilitySystem component is attached is the OwnerActor, and the Actor that is used to represent the physical entity of the AbilitySystem component is typically the AvatarActor.The OwnerActor and AvatarActor can be the same or different.
Following the UE Gameplay framework, the Pawn represents the body and the Controller represents the soul; switching to the GAS framework, the AvatarActor is the body and the OwnerActor is the soul.
If you're new to GAS, or unfamiliar with UE's network framework, I'd suggest that you don't learn from the experts about separating the soul and body just yet. Learn GAS with the flesh and soul aligned (keeping the OwnerActor and AvatarActor the same) first.
For the most part, in your game code, you are getting references to AvatarActor and executing the relevant logic on it. You can explore situations where the OwnerActor and AvatarActor are not the same once you get the hang of GAS.
Grant Ability, AttributeSet and Effect
An AbilitySet is a Data Asset used to configure a set of GameplayAbility, GameplayEffect, and AttributeSet that needs to be given to a character.
AbilitySet is the primary way to give character abilities and effects in a game.
Creating AbilitySet
You create a new ability set by creating a Data Asset of type GGA_AbilitySet(Not subclass from GGA_AbilitySet class).

This picture is an example of a ability set configuration (taken from my combat system)
Usually in a game, a character will have few ability sets, and also replace or add new ability sets based on other game mechanics (e.g. equipment changes, weapon switching) while the game is running.
It is also recommended that you divide different abilities and effects into different ability sets to deal with prioritization issues and for ease of management.
Add/remove AbilitySet at runtime
In addition to adding available AbilitySets to the default settings of Ability System Component , you can also add AbilitySets at runtime.

In my combat system, I give characters corresponding AbilitySets based on whether the weapon is activated or not in the weapon instance.
Tips on granting default ability set
Normally, I will prepare two ability sets. And all of these ability sets will add to the ability system component in order. So you should think carefully about the dependence order.
For example: You should not try to grant a Gameplay Effect to init your attribute before you actually add the attribute set.

One is for configuring something that is required, such as attribute sets the character will use, and I would add "Required" as suffix to this ability set.

Then, I will have another ability set which will grant gameplay effect to initialize my attributes and I normally will add "Default" as suffix to this ability set.
The following image is an example of how to initialize gameplay attributes via GE.

Pay attention to modifier's order, Max attribute comes first.
Ability System Globals
GGA also provided a custom version of AbilitySystemGlobals class.
It provides additional features that allow you to respond to some global event happening inside the ability system.
Global Event Receiver
In native ability system globals, there are some events that are very useful but not exposed to BP and are not easy to use even in C++.
Such as this one:

Implementing interface
Luckily, with GGA, you can have your Actor/Component implement an Interface called "IGGA_AbilitySystemGlobalsEventReceiver".
Make your component implement this interface. When begin/end play, you can register this component to receive events from "ability system globals".

For a combat game, you could implement this interface on your combat component.
Implementation example
When you overriding PreGameplayEffectSpecApply, you can inject some tags based on your game logic, and these tags will affect the subsequent gameplay effect application process.

For example, you can perform a different damage calculation process in Modifier Magnitude Calculation or Gameplay Effect Execution Calculation by determining if SourceTags contain certain Tags.
Stamina related calculations will be enabled and stamina damage will be applied only if the SourceTags contains Impact.Result.Block.
This is how I implement multiple combat calculation flows like "Evading","Dodging","Deflecting" etc.
Global Ability System
There is a WorldSubSystem called "GlobalAbilitySystem" and it's useful when you want to make changes to all ability system component exists in current world.
This system requires you to use GGA_AbilitySystemComponent, and each of these components will be registered to the global ability system when it gets initialized.

For example: If you are making a multiplayer shooting game, you can assign GE_DamageImmune to all players in warm-up phase before the match begins.
Do not confuse it with AbilitySystemGlobals, which is a singleton for internal use only.
Blueprint node extentions
GGA extends GAS a lot, many APIs can be found in the ContextActionList in the blueprint, you can type “GGA|” to filter out all available actions belonging to GGA.

Check out the Blueprint Node List for more details.