Attribute Set Code Generation
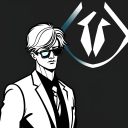
Overview
GGA also has another code module called GenericGameplayAttribute, which is responsible for expanding the GameplayAttribute part of the GAS system and includes some built-in GameplayAttributes. This module is very lightweight.
Attribute and Tag Mapping
GAS very much embraces GameplayTags, however, GameplayAttribute is not driven by GameplayTag, for this reason, GGA provides an optional mapping of GameplayAttribute to GameplayTag.

You can get Attribute through Tag and you can get Tag through Attribute.
You can also register Attribute to a Tag at runtime (this operation is global).
Built-in Attributes
The GenericGameplayAttributes
module comes with three commonly used attribute sets, AS_Health, AS_Mana, and AS_Stamina.

You can use it or not. It is abstract enough and is automatically generated (see below).
How to design an AttributeSet
If you refer to various examples and tutorials on the web, you will find that people are used to using one AttributeSet to define all the attributes used in the game, and they will write a lot of gameplay logic in the AttributeSet, which I don't think is desirable.
Instead, I think AttributeSet should be divided according to the function in the game, and just play the role of data definition. It doesn't need to contain gameplay logic, and only needs to be processed externally according to the logic of attribute changes.
Assuming your game requires two attributes, Health, and Stamina, you should define two AttributeSets.
AS_Health(attributes related to health)
Health | Current value |
MaxHealth | Max value |
Healing | Value to add |
Damage | Value to reduce |
AS_Stamina(attributes related to stamina)
Stamina | Current value |
MaxStamina | Max value |
Healing | Value to add |
Damage | Value to reduce |
The advantages of doing this are:
- In multiplayer games, different characters may need different attributes. In order to avoid a lot of network replication costs, you should only give the set of attributes needed by the characters that need it.
- At the same time, this modular design can go far in the future. (For example, if you use GameFeature, and one of your subFeatures defines its dedicated set of attributes)
Don't abuse GameplayAttributes. Here's a great post that teaches you how to use a small number of attributes and accomplish complex tasks.
Adding new attributes (code generator)
Even for C++ users, adding new attributes can be a very tedious process that requires a lot of code to be written by hand.
GGA provides an additional command line tool that allows you to generate C++ AttributeSet code for you by writing simple Json.
You don't need to write the code by hand, you just need to convert your project into a code project.
Join my Discord server and go to #GGA-Resources to download the Code Generator.
Video Demo
Generation Example
Here is an example of generating gameplay attribute code from json.
1{2 "ModuleName": "GENERICGAMEPLAYATTRIBUTES",3 "ClassName": "AS_Combat",4 "EnableGGA": true,5 "TagPrefix": "GGF.Attribute.CombatSet.",6 "Category": "Attribute|CombatSet",7 "FileName": "AS_Combat",8 "CopyRight": "// Copyright 2024 https://yuewu.dev/en All Rights Reserved.",9 "HeaderOutputDirectory": "E:/GenericGame5.4/Plugins/GenericGameplayAbilities/Source/GenericGameplayAttributes/Public/Attributes",10 "CppOutputDirectory": "E:/GenericGame5.4/Plugins/GenericGameplayAbilities/Source/GenericGameplayAttributes/Private/Attributes",11 "HeaderIncludes": [],12 "CppIncludes": [13 "Attributes/AS_Combat.h"14 ],15 "Attributes": [16 {17 "Name": "Damage",18 "Default": 0,19 "Comment": "The damage that will apply to target"20 },21 {22 "Name": "DamageNegation",23 "Default": 0,24 "Comment": "The damage reduction(percentage) for incoming health damage"25 },26 {27 "Name": "StaminaDamage",28 "Default": 0,29 "Comment": "The stamina damage that will apply to target"30 },31 {32 "Name": "StaminaDamageNegation",33 "Default": 0,34 "Comment": "The damage reduction(percentage) for incoming stamina damage"35 },36 {37 "Name": "PostureDamage",38 "Default": 0,39 "Comment": "The posture(stance) damage that will apply to target"40 },41 {42 "Name": "PostureDamageNegation",43 "Default": 0,44 "Comment": "The damage reduction(percentage) for incoming posture damage"45 }46 ]47}
The Json above generates the following code:
1// Copyright 2024 https://yuewu.dev/en All Rights Reserved.23#pragma once45#include "CoreMinimal.h"6#include "AttributeSet.h"7#include "AbilitySystemComponent.h"8#include "NativeGameplayTags.h"910#include "AS_Combat.generated.h"1112namespace AS_Combat13{1415 GENERICGAMEPLAYATTRIBUTES_API UE_DECLARE_GAMEPLAY_TAG_EXTERN(Damage)1617 GENERICGAMEPLAYATTRIBUTES_API UE_DECLARE_GAMEPLAY_TAG_EXTERN(DamageNegation)1819 GENERICGAMEPLAYATTRIBUTES_API UE_DECLARE_GAMEPLAY_TAG_EXTERN(StaminaDamage)2021 GENERICGAMEPLAYATTRIBUTES_API UE_DECLARE_GAMEPLAY_TAG_EXTERN(StaminaDamageNegation)2223 GENERICGAMEPLAYATTRIBUTES_API UE_DECLARE_GAMEPLAY_TAG_EXTERN(PostureDamage)2425 GENERICGAMEPLAYATTRIBUTES_API UE_DECLARE_GAMEPLAY_TAG_EXTERN(PostureDamageNegation)262728}2930#define ATTRIBUTE_ACCESSORS(ClassName, PropertyName) \31GAMEPLAYATTRIBUTE_PROPERTY_GETTER(ClassName, PropertyName) \32GAMEPLAYATTRIBUTE_VALUE_GETTER(PropertyName) \33GAMEPLAYATTRIBUTE_VALUE_SETTER(PropertyName) \34GAMEPLAYATTRIBUTE_VALUE_INITTER(PropertyName)3536UCLASS()37class GENERICGAMEPLAYATTRIBUTES_API UAS_Combat : public UAttributeSet38{39 GENERATED_BODY()404142public:4344 UAS_Combat();4546 virtual void GetLifetimeReplicatedProps(TArray<FLifetimeProperty>& OutLifetimeProps) const override;4748 virtual void PreAttributeChange(const FGameplayAttribute& Attribute, float& NewValue) override;4950 virtual void PostGameplayEffectExecute(const FGameplayEffectModCallbackData& Data) override;5152 // The damage that will apply to target53 UPROPERTY(BlueprintReadOnly, ReplicatedUsing = OnRep_Damage, Category = "Attribute|CombatSet", Meta = (AllowPrivateAccess = true))54 FGameplayAttributeData Damage{ 0.0 };55 ATTRIBUTE_ACCESSORS(ThisClass, Damage)5657 // The damage reduction(percentage) for incoming health damage58 UPROPERTY(BlueprintReadOnly, ReplicatedUsing = OnRep_DamageNegation, Category = "Attribute|CombatSet", Meta = (AllowPrivateAccess = true))59 FGameplayAttributeData DamageNegation{ 0.0 };60 ATTRIBUTE_ACCESSORS(ThisClass, DamageNegation)6162 // The stamina damage that will apply to target63 UPROPERTY(BlueprintReadOnly, ReplicatedUsing = OnRep_StaminaDamage, Category = "Attribute|CombatSet", Meta = (AllowPrivateAccess = true))64 FGameplayAttributeData StaminaDamage{ 0.0 };65 ATTRIBUTE_ACCESSORS(ThisClass, StaminaDamage)6667 // The damage reduction(percentage) for incoming stamina damage68 UPROPERTY(BlueprintReadOnly, ReplicatedUsing = OnRep_StaminaDamageNegation, Category = "Attribute|CombatSet", Meta = (AllowPrivateAccess = true))69 FGameplayAttributeData StaminaDamageNegation{ 0.0 };70 ATTRIBUTE_ACCESSORS(ThisClass, StaminaDamageNegation)7172 // The posture(stance) damage that will apply to target73 UPROPERTY(BlueprintReadOnly, ReplicatedUsing = OnRep_PostureDamage, Category = "Attribute|CombatSet", Meta = (AllowPrivateAccess = true))74 FGameplayAttributeData PostureDamage{ 0.0 };75 ATTRIBUTE_ACCESSORS(ThisClass, PostureDamage)7677 // The damage reduction(percentage) for incoming posture damage78 UPROPERTY(BlueprintReadOnly, ReplicatedUsing = OnRep_PostureDamageNegation, Category = "Attribute|CombatSet", Meta = (AllowPrivateAccess = true))79 FGameplayAttributeData PostureDamageNegation{ 0.0 };80 ATTRIBUTE_ACCESSORS(ThisClass, PostureDamageNegation)8182 UFUNCTION(BlueprintCallable,BlueprintPure,meta=(DisplayName="GetDamageAttribute"), Category = "Attribute|CombatSet")83 static FGameplayAttribute Bp_GetDamageAttribute();8485 UFUNCTION(BlueprintPure,meta=(DisplayName="GetDamage"), Category = "Attribute|CombatSet")86 float Bp_GetDamage() const;8788 UFUNCTION(BlueprintCallable,meta=(DisplayName="SetDamage"), Category = "Attribute|CombatSet")89 void Bp_SetDamage(float NewValue);9091 UFUNCTION(BlueprintCallable,meta=(DisplayName="InitDamage"), Category = "Attribute|CombatSet")92 void Bp_InitDamage(float NewValue);9394 UFUNCTION(BlueprintCallable,BlueprintPure,meta=(DisplayName="GetDamageNegationAttribute"), Category = "Attribute|CombatSet")95 static FGameplayAttribute Bp_GetDamageNegationAttribute();9697 UFUNCTION(BlueprintPure,meta=(DisplayName="GetDamageNegation"), Category = "Attribute|CombatSet")98 float Bp_GetDamageNegation() const;99100 UFUNCTION(BlueprintCallable,meta=(DisplayName="SetDamageNegation"), Category = "Attribute|CombatSet")101 void Bp_SetDamageNegation(float NewValue);102103 UFUNCTION(BlueprintCallable,meta=(DisplayName="InitDamageNegation"), Category = "Attribute|CombatSet")104 void Bp_InitDamageNegation(float NewValue);105106 UFUNCTION(BlueprintCallable,BlueprintPure,meta=(DisplayName="GetStaminaDamageAttribute"), Category = "Attribute|CombatSet")107 static FGameplayAttribute Bp_GetStaminaDamageAttribute();108109 UFUNCTION(BlueprintPure,meta=(DisplayName="GetStaminaDamage"), Category = "Attribute|CombatSet")110 float Bp_GetStaminaDamage() const;111112 UFUNCTION(BlueprintCallable,meta=(DisplayName="SetStaminaDamage"), Category = "Attribute|CombatSet")113 void Bp_SetStaminaDamage(float NewValue);114115 UFUNCTION(BlueprintCallable,meta=(DisplayName="InitStaminaDamage"), Category = "Attribute|CombatSet")116 void Bp_InitStaminaDamage(float NewValue);117118 UFUNCTION(BlueprintCallable,BlueprintPure,meta=(DisplayName="GetStaminaDamageNegationAttribute"), Category = "Attribute|CombatSet")119 static FGameplayAttribute Bp_GetStaminaDamageNegationAttribute();120121 UFUNCTION(BlueprintPure,meta=(DisplayName="GetStaminaDamageNegation"), Category = "Attribute|CombatSet")122 float Bp_GetStaminaDamageNegation() const;123124 UFUNCTION(BlueprintCallable,meta=(DisplayName="SetStaminaDamageNegation"), Category = "Attribute|CombatSet")125 void Bp_SetStaminaDamageNegation(float NewValue);126127 UFUNCTION(BlueprintCallable,meta=(DisplayName="InitStaminaDamageNegation"), Category = "Attribute|CombatSet")128 void Bp_InitStaminaDamageNegation(float NewValue);129130131 UFUNCTION(BlueprintCallable,BlueprintPure,meta=(DisplayName="GetPostureDamageAttribute"), Category = "Attribute|CombatSet")132 static FGameplayAttribute Bp_GetPostureDamageAttribute();133134 UFUNCTION(BlueprintPure,meta=(DisplayName="GetPostureDamage"), Category = "Attribute|CombatSet")135 float Bp_GetPostureDamage() const;136137 UFUNCTION(BlueprintCallable,meta=(DisplayName="SetPostureDamage"), Category = "Attribute|CombatSet")138 void Bp_SetPostureDamage(float NewValue);139140 UFUNCTION(BlueprintCallable,meta=(DisplayName="InitPostureDamage"), Category = "Attribute|CombatSet")141 void Bp_InitPostureDamage(float NewValue);142143 UFUNCTION(BlueprintCallable,BlueprintPure,meta=(DisplayName="GetPostureDamageNegationAttribute"), Category = "Attribute|CombatSet")144 static FGameplayAttribute Bp_GetPostureDamageNegationAttribute();145146 UFUNCTION(BlueprintPure,meta=(DisplayName="GetPostureDamageNegation"), Category = "Attribute|CombatSet")147 float Bp_GetPostureDamageNegation() const;148149 UFUNCTION(BlueprintCallable,meta=(DisplayName="SetPostureDamageNegation"), Category = "Attribute|CombatSet")150 void Bp_SetPostureDamageNegation(float NewValue);151152 UFUNCTION(BlueprintCallable,meta=(DisplayName="InitPostureDamageNegation"), Category = "Attribute|CombatSet")153 void Bp_InitPostureDamageNegation(float NewValue);154155protected:156157 /** Helper function to proportionally adjust the value of an attribute when it's associated max attribute changes. (i.e. When MaxHealth increases, Health increases by an amount that maintains the same percentage as before) */158 virtual void AdjustAttributeForMaxChange(FGameplayAttributeData& AffectedAttribute, const FGameplayAttributeData& MaxAttribute, float NewMaxValue, const FGameplayAttribute& AffectedAttributeProperty);159160 UFUNCTION()161 virtual void OnRep_Damage(const FGameplayAttributeData& OldValue);162163 UFUNCTION()164 virtual void OnRep_DamageNegation(const FGameplayAttributeData& OldValue);165166 UFUNCTION()167 virtual void OnRep_StaminaDamage(const FGameplayAttributeData& OldValue);168169 UFUNCTION()170 virtual void OnRep_StaminaDamageNegation(const FGameplayAttributeData& OldValue);171172 UFUNCTION()173 virtual void OnRep_PostureDamage(const FGameplayAttributeData& OldValue);174175 UFUNCTION()176 virtual void OnRep_PostureDamageNegation(const FGameplayAttributeData& OldValue);177};
As you can see, just a simple set of attributes requires so much code. So code generation is given!
Generated Bluepirnt Nodes



A guide to the code generator
It's just a simple executable (gga.exe) written in Golang. Users only need to know about how to use it (Tools's source code will available soon), the code it generates is code after all, and if you're not happy with it you can still modify it.
Convert project to C++
Make sure to convert the project into a C++ project and add plugin related dependencies, I will add a more detailed tutorial about this part later.
Defining json and generating the code
Let's assume you have a directory containing gga.exe, and one configured Json.

The ‘ModuleName’ must be in all capitals, and don't forget to add ‘EnableGGA’: true.
Simply open CMD (Win10) or Terminal (Win11) in the directory where gga.exe is located and type in the following commands.
./gga.exe gen -f ./Sample.json
Then you should recompile your project.
Note: . / is the current path, gga.exe is the generator, gen means that the operation is a generate operation, and the -f option specifies the file to be generated. The -f option specifies the file to be generated, and /xxx.json is the actual property definition.
Modify Code generation template

As you can see, these two files are templates used to generate code. You can also modify it to add additional code (Just don't break the if/else/for in template files. )
Future plans to code generator
I have a plan to integrate code generator into unreal editor, You can configure your attribute set with data table and generate code via asset action.


Some thoughts
Since the attribute system of GAS has to be done in C++, this has hindered a large portion of blueprint users, while various solutions for blueprint users have appeared in Unreal Marketplace. Even a pure blueprint GameplayAttribute solution appeared.
However, I think that if you take a big detour to use GameplayAttribute with all kinds of hacks just because you have a fear of code projects, I think you are putting the cart before the horse.
What if in the future Unreal comes with blueprint support for GameplayAttribute?
What if in the future the Unreal team makes it so that GAS no longer requires C++?
Of course, this is just my own personal opinion. If you're going to develop serious games, you're always going to be faced with C++.