Gameplay Ability Basic
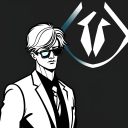
GameplayAbilities
Check https://github.com/tranek/GASDocumentation?tab=readme-ov-file#46-gameplay-abilities to learn the basics of gameplay abilities.
Gameplay Ability Definition
In the game world, many actions that an actor can perform can be realized through Gameplay Abilities (GA). You can create new abilities by inheriting from GameplayAbility
or GGA_GameplayAbility
using Blueprints/C++.
You can override the corresponding functions to implement the relevant functionalities of the abilities.
Simple Ability Example (Jump)

This abilities allows the character to jump when activated. Upon ability end, it stops the jump. Since this ability does not store any state or data and only executes logic, its instancing policy can be set to NonInstanced (meaning no instance of ability class is created).

Additionally, it overrides the CanActivateAbility
function to check whether the current character is able to jump.
Ability Tags
Check here: https://github.com/tranek/GASDocumentation?tab=readme-ov-file#469-ability-tags
Understanding Ability Definitions and Instances
First, there are a few concepts I need to clarify, as they can be quite confusing. Even C++ users often struggle to understand the relationships between them.
- Ability Definition: Any Blueprint asset created in the editor that inherits from
GameplayAbility
, or any subclass ofGameplayAbility
created in C++, can be understood as an ability definition. - Ability Instance: The struct
GameplayAbilitySpec
, created when an ability definition is granted to anAbilitySystem
component, can be understood as an ability instance. - Ability Definition Instance: Since an ability definition is a class, the instantiated object of that class is referred to as an ability definition instance. The creation rules for ability definition instances are determined by the instancing policy defined within the ability definition itself.

Ability Instances
When an ability is granted to an AbilitySystem
component, a struct called GameplayAbilitySpec
is created, which you can think of as the ability instance. This structure contains a wealth of information about the ability, such as the definition class used during its creation, its level, and more.
Additionally, GAS provides a lightweight struct called GameplayAbilitySpecHandle
, which can be understood as a "pointer" to the ability instance. In actual development, it is common to pass the SpecHandle
around in various code executions to avoid directly passing the AbilitySpec
, which is heavy to copy around.
The APIs within GameplayAbilitySpec
are hidden in C++, but GGA provides functions to access information within the spec. In most cases, Blueprint users only need to operate using the AbilitySpecHandle
.
Ability Instancing Policy
In the ability definition, you can choose from three different instancing policies:

- Non-Instanced
Don't use it as this policy has been deprecated since UE5.5. - Instanced Per Actor
This policy creates an instance of the ability definition for each actor when the ability definition is granted to ability system component. This is the most common and aligns well with the design of GAS, meaning each actor has its own unique copy of the ability. - Instanced Per Execution
This policy creates a new instance every time the ability is activated. In practical project development, this is very resource-intensive and rarely used. If you are a beginner, it's advisable to avoid this policy.
How to Choose
In most cases, you should use Instanced Per Actor
to keep things simple and avoid potential issues.
Retrieving Ability Instances
Once an ability is granted to the AbilitySystem
component, it is added to an internal list of ability instances maintained by the component. When you need to perform operations on an ability, you must first retrieve the specific ability instance you wish to work with.
GAS provides default functions to obtain the ability instances:

However, GGA offers additional convenient functions for accessing ability instances:

Activating Ability Instances
Here are the main methods for activating an ability:

It is advisable to avoid activating abilities through their class definitions, as this approach lacks flexibility. Doing so couples your code to specific ability definitions and loses the polymorphism provided by Gameplay Tags.
For example, if you have two different melee AIs that activate an attack through the tagLightAttack
, they may each have their own unique version ofLightAttack
.
You can also use the following API to check whether a specific ability can be activated:

Cancelling Ability Instances
In addition to calling EndAbility
from within the ability itself, you can also cancel abilities externally:

Retrieving Ability Definition Instances
Depending on the instancing policy of the ability definition, once you have an ability instance, you can choose the appropriate API to retrieve the ability definition instance:
